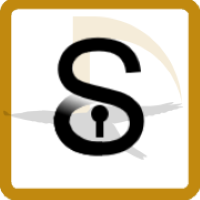
Scriptlock 2.1
The code execution prevention system for JavaScript
Demonstration
The best way to prove the effectiveness of Scriptlock is to show it in action.
Scriptlock provides fully deadlocked protection in IE 10, IE11, Edge 12+, Safari 6 +, Chrome 18.0+, Firefox 6.0 +, Opera 10.5+. So if you are using or have access to any of these browsers then you will be able to see the different protection mechanisms provided by Scriptlock at work.
There are broadly three protection mechanism that Scriptlock applies depending on the browser you are using:
- Pre-CSP protection
- CSP1.0 protection
- CSP2.0 protection
The following table shows which browser versions you will need to be using for each protection mechanisms. There
are still reference copies of all versions of Firefox readily available from the Mozilla website. IE11 is still shipped with Windows 10 and
accessible from the run prompt iexplore.exe
.
Mechanism | Browser Make | Version |
---|---|---|
pre-CSP | Internet Explorer | 10 - 11 |
pre-CSP | Firefox* | 6 - 22 |
pre-CSP | Safari* | 6 |
pre-CSP | Chrome* | 18-24 |
pre-CSP | Opera | 10.5-14 |
CSP 1.0 | Edge | 12 - 14 |
CSP 1.0 | Firefox | 23 - 30 |
CSP 1.0 | Safari | 7 - 9.1 |
CSP 1.0 | Chrome | 25 - 35 |
CSP 1.0 | Opera | 15-22 |
* these versions do support CSP1.0 but use non-standard headers.
Persistent-XSS protection
The following series of tests that demonstrate Scriptlock's abilities to protect agains Persistent XSS and Reflected XSS and variable hijacking. They also demonstrate a number of other features:
- The 'inline-event' source, along with the event-nonce and the comment based nonce.
- Script synchronisation in the CSP1.0 mechanism.
- The effectiveness of Scriptlock's call-stack based algrotihm in the pre-CSP protection mechanism.
Note an event listener is added to each button to translate a "no action" outcome to a pass or fail as appropriate. You will therefore notice subtle differences in the results of tests between the pre-CSP protection mechanism, the CSP 1.0 mechanism and CSP 2.0. For instance Test 6 will return "No action (Pass)" with the first and "Pass" with the other two. This is entirely correct behaviour.
For each test bring up the F12 debugger to see the protection mechanisms kicking in.
Test | Details | Button | Result |
---|---|---|---|
Test 1 |
Inline event on an element with no nonce specifed
|
||
Test 2 |
Inline event on an element with a valid event-nonce attribute
|
||
Test 3 |
Inline event on an element with a valid comment based nonce
|
||
Test 4 |
Applying an inline function with an addEventListener call from a timeout invoked from within the scope of an inline script without a valid nonce.
|
||
Test 5 |
Variable hijacking. addEventListener called from within the scope of an allowed inline script, inadvertantly applying a bad inline function.
|
||
Test 6 |
Variable hijacking. addEventListener called the scope of an allowed inline script, inadvertantly applying a bad inline function via a timeout.
|
||
Test 7 |
Variable hijacking. addEventListener called in the scope of an allowed inline script, inadvertantly applying a bad inline function defined in an external script.
|
||
Test 8 |
addEventListener called in the scope of an allowed inline script, applying an anonymous function.
|
||
Test 9 |
addEventListener called in the scope of an allowed inline script, applying an inline function defined in an allowed script.
|
||
Test 10 |
addEventListener called in the scope of a timeout spawned from an allowed inline script, applying an inline function defined in an allowed script.
|
||
Test 11 |
Variable hijacking. Inadvertantly setting onclick property to a bad inline function in the scope of an allowed inline script
|
||
Test 12 |
Variable hijacking. Inadvertantly setting onclick property to a function defined in a bad external script in the scope of an allowed inline script.
|
||
Test 13 |
Setting onclick property to an anonymous function in the scope of an allowed inline script.
|
||
Test 14 |
Setting onclick property to an inline function, defined in an allowed script, from within the scope of an allowed inline script.
|
||
Test 15 |
Asynchronous side-loading of script with an onloaded event (In this case the external script sets parameter_for_test_15 = 15).
|